If we want to store values like name, age, and date in computer memory, we need access to memory addresses. Since working with a memory address is complicated, high-level languages like Java provide us an easy way to refer them by using variables.
Variable is a named memory location where you can store values. The data in a variable can vary from time to time, that’s why it is called variable.
Declaration of variable
Java is a strongly typed language. To use any variable in a Java program, you need to declare the variable with a data type.
int age;
Here, age is being declared as integer variable, that is, age variable stores only integers.
Initializing a variable
A variable can be initialized after declaration, or both declaration and initialization can be written in a single statement also.
int age; // declaration
age = 30; // initialization
int count = 5; // declaration and initialization
Rules for naming a variable
- Variable name should not start with a number
- alphabets, numbers, $ and _ are allowed characters in a variable name
Naming Conventions
- Variable name to start with lower case letter
- If variable contains two words, second word first letter to be capital letter Ex:
firstName
Using a Variable
Before retrieving value from a variable, it must be initialized with some value.
int a;
int b;
b = a; // compilation error
int a = 0;
int b;
b = a; // this is good, a and b both will be 0
Types of Variables
There are two types of variables: (1) Primitive Variables (2) Reference Variables
Primitive Variables
Primitive variables are used with primitive values. Primitive variables store values directly at the allocated variable memory location.
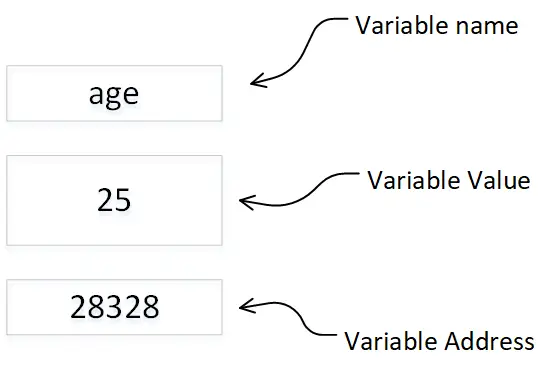
The assignment operation between two variables copies the value of one variable into another. After the assignment operation, both variables will have the same duplicated value at their memory location.
Reference Variable
- Reference variables are used for storing objects.
- Both the reference variable and object will have their unique memory addresses.
- Reference variable holds the address of an object
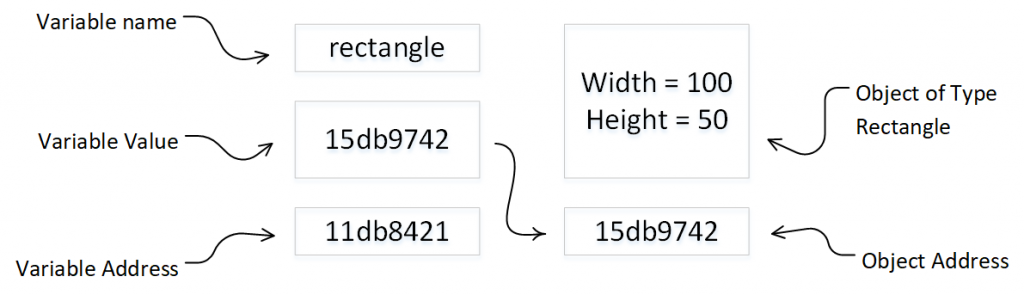
When you perform assignment operation between two reference variables, object address will be copied to another variable. So, both reference variables will be having same value that is object’s address. Since both reference variables have same object address value, they both point to the same object.
String a = new String("Hello");
String b = a;
In this code snippet, we are creating an object using new String("Hello")
. The new object address gets stored in variable a. When we assign a to b, object address copied into b as well. Now, both a and b are pointing to the same “Hello” object.