An if-else statement conditionally execute one or more statement. It contains 3 parts, 1) condition 2) true block 3) else block.
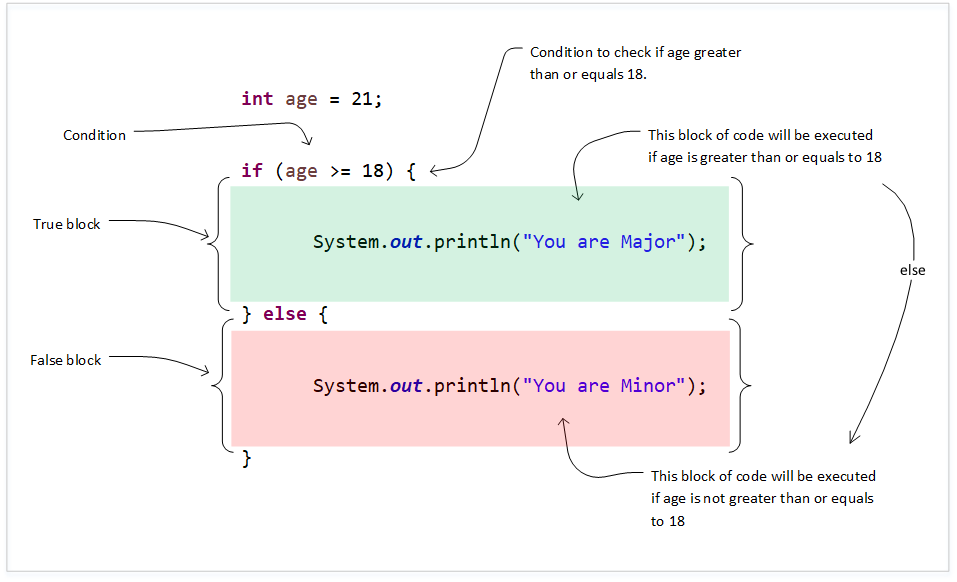
if-else condition example
package com.techstackjournal;
public class IfCondition {
public static void main(String[] args) {
int age = 21;
if (age >= 18) {
System.out.println("You are Major");
} else {
System.out.println("You are Minor");
}
}
}
You are Major
if-else condition without brackets
int a = 5, b = 10;
if(a > b)
System.out.println("a is greater than b");
System.out.println("b is smaller than a");
System.out.println("end of program.");
b is smaller than a
end of program.
Java allows you to write if-else statement without brackets. But in this case, only the statement immediately after the if condition will be within used for condition.
In the above code snippet, only “a greater than b” is within the if condition, “b is smaller than a” is not within if condition, as we didn’t wrapped it inside a block using brackets.
It is always good practice to include brackets, no matter what the number statements inside the condition. It makes code readable.
if – else if – else statement
Say, you want to check the age and print Senior Citizen, Major or Minor according to the age. Below is the wrong way of doing it, as it checks 2 more conditions, even if the first one is successful.
// Wrong way of checking multiple conditions
package com.techstackjournal;
public class MultipleConditions {
public static void main(String[] args) {
int age = 65;
if (age >= 60) {
System.out.println("You are Senior Citizen");
}
if (age >= 18) {
System.out.println("You are Major");
}
if (age < 18) {
System.out.println("You are Minor");
}
}
}
The correct way of checking these conditions is by chaining if statements (also called as multi-level), using if – else if – else statement. This way, if the first condition is successful, it will not check other conditions further.
package com.techstackjournal;
public class IfElseIfElseStatement {
public static void main(String[] args) {
int age = 65;
if (age >= 60) {
System.out.println("You are Senior Citizen");
} else if (age >= 18) {
System.out.println("You are an Major");
} else {
System.out.println("You are an Minor");
}
}
}
You are Senior Citizen
Nested if statements
Writing if statement within another if statement is called nesting of if statements.
package com.techstackjournal;
public class NestedIfCondition {
public static void main(String[] args) {
int age = 35;
if (age >= 18) {
System.out.println("Adult");
if (age > 60) {
System.out.println("Senior Citizen");
}
if (age > 30) {
System.out.println("Middle aged");
}
} else {
System.out.println("Minor");
}
}
}
Adult
Middle aged
It is always advised to limit the depth of the nested-if to 1 or max 2, otherwise code will lose its readability.
Blocks and Variable Scope
In Java, a block is represented by beginning and curly brackets. So far, we have seen class
, main
method and if
condition have blocks. (We will discuss classes in later modules.)
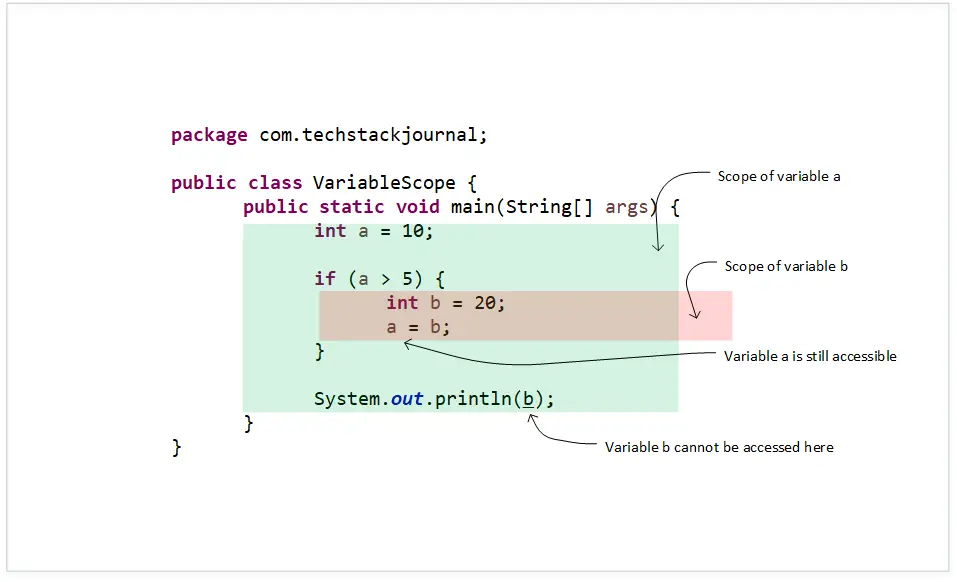
Each block has its own variable scope, that is, a variable declared within a block is accessible only within that block, and not outside of it.
Variables that are already in-scope when the block starts, they remain in-scope. But variables that are declared within the block will go out-of-scope at the end of the block.