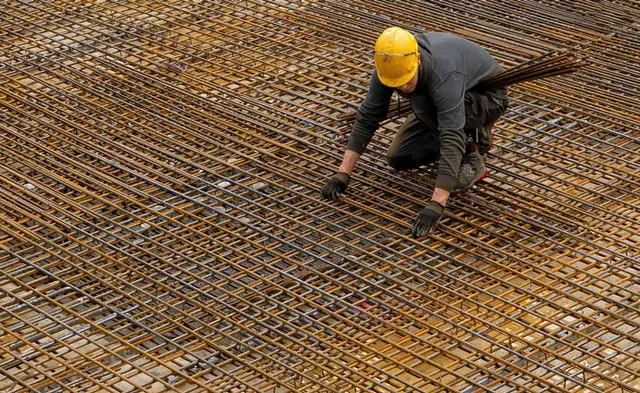
Definition
A Constructor is a block of code which gets executed by Java when you create an instance of a class. Constructor looks similar to a function. But they do not have a return type. The name of a constructor must be a class name.
If I’m creating an Employee class, I can write the constructor as:
public Employee() {
// Code
}
A Very Simple Example of a Constructor
package com.techstackjournal;
public class Employee {
public Employee() {
System.out.println("In Employee() Constructor...");
}
public static void main(String args[]) {
Employee emp = new Employee();
}
}
In this class, we just have a constructor. It is printing a simple message. I’m creating an instance of this class in the main method. That’s it, I’m not calling any method on that reference variable. The output of this program will be as below.
In Employee() Constructor...
How that message got printed without calling the constructor? We do not call the constructor. Java interpreter will call the constructor while instantiating the class. Since we are creating an object without passing arguments by writing new Employee()
, Java will search if there is a constructor without arguments and executes it.
Default Constructor
In Java, every class will have a constructor. If you do not write a constructor, Java will insert one empty constructor for you. We call this empty constructor as a default constructor. A default constructor doesn’t contain any arguments or code. If you write at least one constructor, this automatic insertion of default constructor doesn’t happen.
Constructor with Arguments
Since constructor is the first thing that gets executed when we create an object, it is the best place to initialize the object.
Constructor can have arguments, but it is not a function, so it will not have a return statement.
package com.techstackjournal.app.types;
public class Employee {
private String id;
private String name;
private double salary;
public Employee(String id, String name, double salary) {
this.id = id;
this.name = name;
this.salary = salary;
}
public String toString() {
return "Employee [id=" + id + ", name=" + name + ", salary=" + salary + "]";
}
}
In this class, we’ve one Employee constructor which takes multiple variables as constructor arguments, which are being used for initializing the instance variables.
Here, id, name and salary are constructor arguments or just variables. However, this.id, this.name and this.salary are the instance variables.
Keyword this
always refers to the current object.
Keyword this
is not mandatory to refer the instance variables, however, when the argument variable and instance variable both have the same name, we can use this
keyword to differentiate both of them. But it is a general practice to use this
keyword when we are referring to the instance variable.
Let’s create a main program to create an instance of this Employee class using constructor.
package com.techstackjournal.app.main;
import com.techstackjournal.app.types.Employee;
public class Application {
public static void main(String[] args) {
Employee emp = new Employee("E001", "John Doe", 8000);
System.out.println(emp);
}
}
In this main program, we are passing the employee details while creating an instance of Employee class.
We need to make sure the order of the arguments is matching with the definition of the constructor. Since Employee constructor accepts first two arguments as String and the last argument as double, we need to pass the arguments in similar sequence. However, if you pass name first and employee id next, Java cannot warn you anything as for it both of them are Strings.
When there were no constructors in the class, we could create an instance of the class using an empty constructor. But if a class contains at least one constructor with arguments but not an empty constructor, you will not be able to instantiate the class using empty constructor call now.
Employee emp = new Employee();
Above line of code will throw a compilation error as we do not have empty constructor in the class.
Constructor Overloading
Writing more than one constructor in a class with unique set of arguments is called as Constructor overloading. When you have more than one constructor,
package com.techstackjournal.app.types;
public class Employee {
private String id;
private String name;
private double salary;
public Employee() {
System.out.println("Constructing with 1 argument...");
this.salary = 8000;
}
public Employee(String id, String name) {
System.out.println("Constructing with 2 arguments...");
this.id = id;
this.name = name;
}
public Employee(String id, String name, double salary) {
System.out.println("Constructing with 3 arguments...");
this.id = id;
this.name = name;
this.salary = salary;
}
public String toString() {
return "Employee [id=" + id + ", name=" + name + ", salary=" + salary + "]";
}
}
In the above class, there are 3 constructors, a constructor without arguments, a constructor with 2 arguments and a constructor with 3 arguments.
Let’s create a main program to consume this Employee class.
package com.techstackjournal.app.main;
import com.techstackjournal.app.types.Employee;
public class Application {
public static void main(String[] args) {
Employee emp1 = new Employee();
Employee emp2 = new Employee("E001", "John Doe");
Employee emp3 = new Employee("E002", "John Doe", 9000);
System.out.println("Employee 1: " + emp1);
System.out.println("Employee 2: " + emp2);
System.out.println("Employee 3: " + emp3);
}
}
Constructing with 1 argument...
Constructing with 2 arguments...
Constructing with 3 arguments...
Employee 1: Employee [id=null, name=null, salary=8000.0]
Employee 2: Employee [id=E001, name=John Doe, salary=0.0]
Employee 3: Employee [id=E002, name=John Doe, salary=9000.0]
As you can see in the output, creating an object without any arguments will call no-arg constructor, creating an object with 2 arguments calls 2-arg constructor and so on.
Frequently Asked Questions on Constructor in Java
Can constructor be private?
Yes, if you do not want anyone to create an instance of a class, you can declare a constructor as private. To use a class with private constructor, you need to expose its methods by declaring them as static.
What is the difference between class and constructor?
A class is a template, and it contains member variables, member functions, class methods and constructors too. However, a constructor which also has the same name as that of its class is just a piece of code within the class itself. When we instantiate a class, Java automatically calls respective constructor.
When a constructor is called in Java?
A constructor will be called when we instantiate the class using a new keyword. Java not only calls the constructor of just the instantiated class but also of all its superclasses starting from the superclass to subclass. Java executes the constructor after completion of static block and instance block execution.
Can constructor be final?
No, we can only declare a class, method and variable as final. Declaring a constructor as final doesn’t make sense, as a sub-class anyway doesn’t inherit constructors from its parent. Each class has its own constructors.
Can abstract class have constructor?
We can have constructor within an abstract class. We can write the object initialization logic, which is relevant to the attributes declared within that abstract class. But you may ask why we need a constructor when an abstract class cannot be instantiated. It would make sense to initializing the member variables which were defined in the abstract class, so for that we can make use of the constructor.
Though you cannot instantiate an abstract class, we can extend it using a sub-class. When you create an instance of the subclass, Java will execute all the super-class constructors starting from the superclass to subclass.
package com.techstackjournal;
abstract class Alpha {
public Alpha() {
System.out.println("Alpha");
}
}
class Beta extends Alpha {
public Beta() {
System.out.println("Beta");
}
}
public class AbstractClassDemo {
public static void main(String[] args) {
Beta b = new Beta();
}
}
Output:
Alpha
Beta
Can final class have constructor?
Yes, a final class can have a constructor and that constructor will be invoked when you instantiate that final class.
package com.techstackjournal;
final class Beta {
public Beta() {
System.out.println("Beta");
}
}
public class FinalClassDemo {
public static void main(String[] args) {
Beta b = new Beta();
}
}
Output:
Beta