Overview
There are 4 levels of access that you can specify on members of a class using access modifiers. Those are public
, private
, protected
and default. In an earlier section, we have learned about private
and public
access modifiers, we will revisit those once again here. But before that let’s check how much you can recollect.
Learning Check
[wp_quiz id=”947″]
Members of a class declared public
can be accessed outside of the class. However, members that are declared private
can be accessed only within the class.
Apart from these two, we have protected and default access levels, default doesn’t have access modifier keyword.
If you simply declare an instance variable without any access modifier, it will be considered as default access.
In the below image you can see how the instance variables of Alpha class are impacted with different access levels when they are accessed via its objects and subclasses, within the same package and outside of the package.
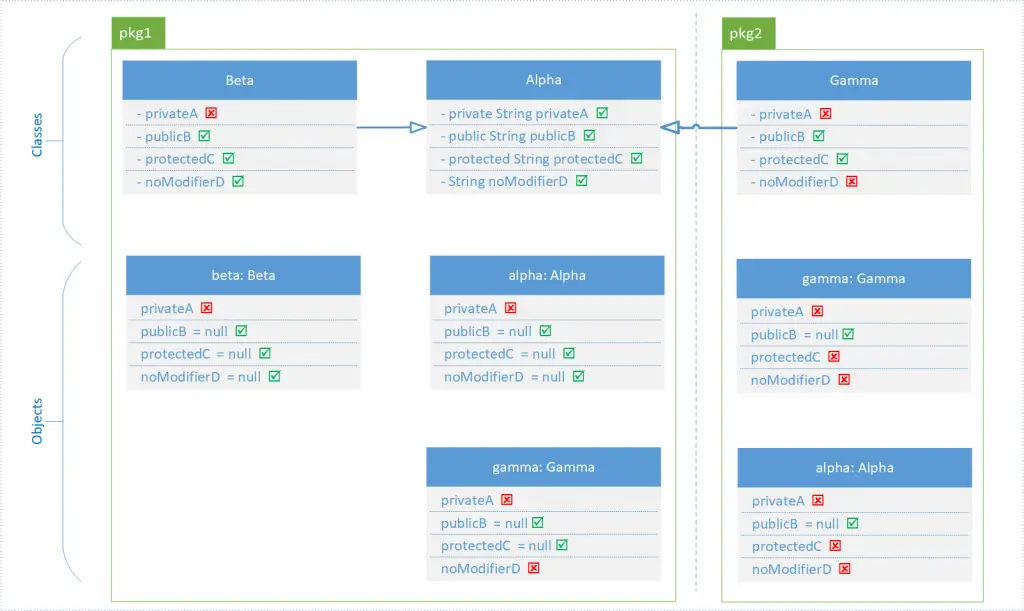
Access Modifier – public
- A member that is declared as
public
is accessible within the class and all of its sub-classes even if those sub-classes are present in other packages. - Also,
public
members are accessible outside of the class in any package through its instance
Access Modifier – protected
- A member that is declared as protected is
- accessible within the class
- accessible within subclass in the same package as the super-class
- accessible outside of the class using dot operator within the same package as of the class
- accessible outside of the subclass using dot operator within the same package as of the super-class
- accessible within a subclass, where subclass present in another package
- not accessible outside of the class using dot operator within another package
- not accessible outside of the subclass using dot operator within another package where a subclass is also present in another package
- accessible by its subclass or subclass’s instance, where subclass present in another package but instantiated in super-class package
Access Modifier – no modifier
- A member that is declared without any modifier is
- accessible within the class
- accessible within subclass in the same package as the super-class
- accessible outside of the class using dot operator within the same package as of the class
- accessible outside of the subclass using dot operator within the same package as of the super-class
- not accessible within a subclass, where subclass present in another package
- not accessible outside of the class using dot operator within another package
- not accessible outside of the subclass using dot operator within another package where a subclass is also present in another package
- accessible outside of the subclass using dot operator, where subclass present in another package but instantiated in super-class package
Access Modifier – private
- A member that is declared as private is only accessible within its class and nowhere else.
Example
All the commented lines in the below programs cause compilation error due to access level restrictions.
package com.techstackjournal.accessmodifiers.pkg1;
public class Alpha {
public String publicA = "public variable is accessible";
private String privateB = "private variable is accessible";
protected String protectedC = "protected variable is accessible";
String noModifierD = "default variable is accessible";
public void display() {
System.out.println("Within the class Alpha " + this.publicA);
System.out.println("Within the class Alpha " + this.protectedC);
System.out.println("Within the class Alpha " + this.noModifierD);
System.out.println("Within the class Alpha " + this.privateB);
}
}
package com.techstackjournal.accessmodifiers.pkg1;
public class Beta extends Alpha {
public void display() {
System.out.println("Within the class Beta " + this.publicA);
System.out.println("Within the class Beta " + this.protectedC);
System.out.println("Within the class Beta " + this.noModifierD);
// System.out.println("Within the class Beta " + this.privateB);
}
}
package com.techstackjournal.accessmodifiers.pkg2;
import com.techstackjournal.accessmodifiers.pkg1.Alpha;
public class Gamma extends Alpha {
public void display() {
System.out.println("Within the class Gamma " + this.publicA);
System.out.println("Within the class Gamma " + this.protectedC);
// System.out.println("Within the class Gamma "+ this.noModifierD);
// System.out.println("Within the class Gamma "+ this.privateB);
}
}
package com.techstackjournal.accessmodifiers.pkg1;
import com.techstackjournal.accessmodifiers.pkg2.Gamma;
public class Pkg1App {
public static void main(String[] args) {
Alpha alpha = new Alpha();
alpha.display();
System.out.println("Outside of Alpha " + alpha.publicA);
System.out.println("Outside of Alpha " + alpha.protectedC);
System.out.println("Outside of Alpha " + alpha.noModifierD);
// System.out.println("Outside of Alpha " + alpha.privateB);
Beta beta = new Beta();
beta.display();
System.out.println("Outside of Beta " + beta.publicA);
System.out.println("Outside of Beta " + beta.protectedC);
System.out.println("Outside of Beta " + beta.noModifierD);
// System.out.println("Outside of Beta " + beta.privateB);
Gamma gamma = new Gamma();
gamma.display();
System.out.println("Outside of Gamma " + gamma.publicA);
System.out.println("Outside of Gamma " + gamma.protectedC);
// System.out.println("Outside of Alpha " + gamma.noModifierD);
// System.out.println("Outside of Alpha " + beta.privateB);
}
}
Within the class Alpha public variable is accessible
Within the class Alpha protected variable is accessible
Within the class Alpha default variable is accessible
Within the class Alpha private variable is accessible
Outside of Alpha public variable is accessible
Outside of Alpha protected variable is accessible
Outside of Alpha default variable is accessible
Within the class Beta public variable is accessible
Within the class Beta protected variable is accessible
Within the class Beta default variable is accessible
Outside of Beta public variable is accessible
Outside of Beta protected variable is accessible
Outside of Beta default variable is accessible
Within the class Gamma public variable is accessible
Within the class Gamma protected variable is accessible
Outside of Gamma public variable is accessible
Outside of Gamma protected variable is accessible
package com.techstackjournal.accessmodifiers.pkg2;
import com.techstackjournal.accessmodifiers.pkg1.Alpha;
import com.techstackjournal.accessmodifiers.pkg1.Beta;
public class Pkg2App {
public static void main(String[] args) {
Alpha alpha = new Alpha();
alpha.display();
System.out.println("Outside of Alpha "+alpha.publicA);
// System.out.println("Outside of Alpha " + alpha.protectedC);
// System.out.println("Outside of Alpha " + alpha.noModifierD);
// System.out.println("Outside of Alpha " + alpha.privateB);
Beta beta = new Beta();
beta.display();
System.out.println("Outside of Beta " + beta.publicA);
// System.out.println("Outside of Beta " + beta.protectedC);
// System.out.println("Outside of Beta " + beta.noModifierD);
// System.out.println("Outside of Beta " + beta.privateB);
Gamma gamma = new Gamma();
gamma.display();
System.out.println("Outside of Gamma " + gamma.publicA);
// System.out.println("Outside of Gamma " + gamma.protectedC);
// System.out.println("Outside of Gamma " + gamma.noModifierD);
// System.out.println("Outside of Gamma " + beta.privateB);
}
}
Within the class Alpha public variable is accessible
Within the class Alpha protected variable is accessible
Within the class Alpha default variable is accessible
Within the class Alpha private variable is accessible
Outside of Alpha public variable is accessible
Within the class Beta public variable is accessible
Within the class Beta protected variable is accessible
Within the class Beta default variable is accessible
Outside of Beta public variable is accessible
Within the class Gamma public variable is accessible
Within the class Gamma protected variable is accessible
Outside of Gamma public variable is accessible
Access Modifiers on Class
- A normal class which is not nested in another class can have only 2 access levels: public and default
- A class that is declared as
public
is accessible by all packages - A class declared without public keyword is accessible by classes which are present in the same package
- A class that is declared as
- A nested class, that is a class within another class, can have all 4 access levels
Access Modifier Quiz
[wp_quiz id=”957″]