Operators are symbols that can be used along with one or two or three operands to produce a result. A operand can be a variable or a literal based on the type of operation and position of the operand. Operand provides required values to operator to perform an operation.
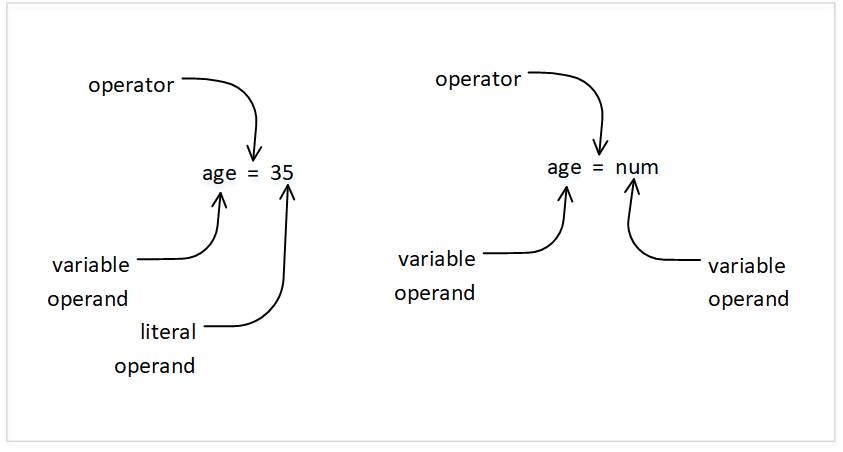
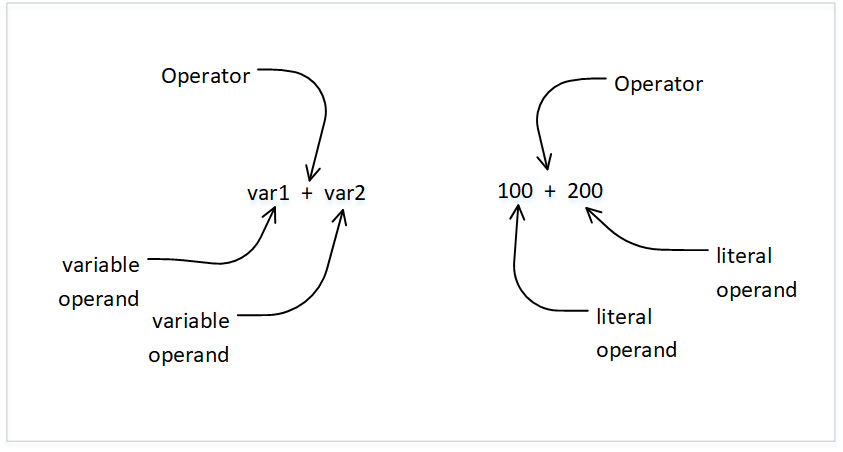
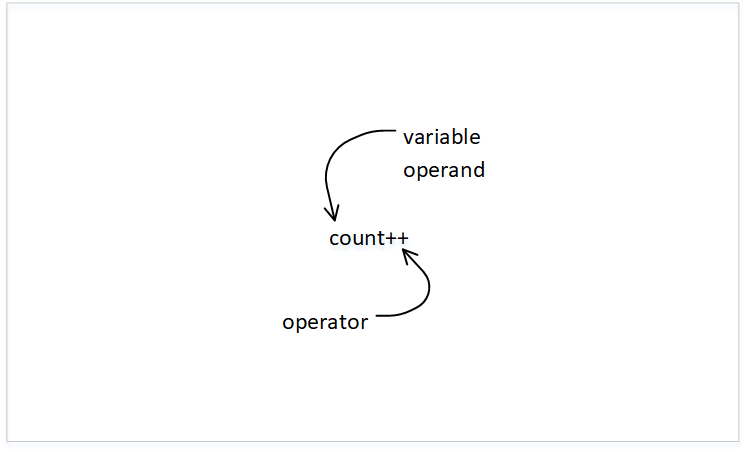
Java provides various operators, such as, Additive Operators, Multiplicative Operators, Assignment Operators, Unary Operators, Bitwise Operators, Relational Operators, Equality Operators and Conditional Operators.
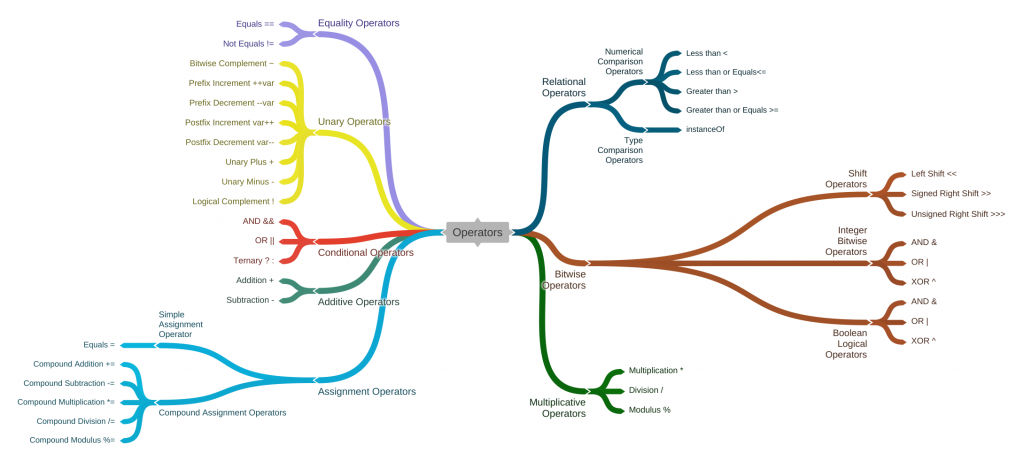
In the above mind map we depicted all of Java operators. However, for easiness we will group them at high-level as Arithmetic Operators, Bitwise Operators, Equality & Relational Operators and Logical & Conditional Operators in the next few sections.
Arithmetic Operators
Arithmetic operators can be further grouped into basic operators, unary operators, compound operators.
Basic Operators
Operation | Operator |
---|---|
Addition | + |
Subtraction | – |
Multiplication | * |
Division | / |
Modulus | % |
Basic operations on integer Numbers
int v1 = 5 + 2; // 7
int v2 = 5 - 2; // 3
int v3 = 5 * 2; // 10
int v4 = 5 / 2; // 2
int v5 = 5 % 2; // 1
Basic Operations on Floating Point Numbers
double d1 = 5 + 2; // 7.0
double d2 = 5 - 2; // 3.0
double d3 = 5 * 2; // 10.0
double d4 = 5 / 2; // 2.5
double d5 = 5 % 2; // 1.0
Unary Operators
Unary operators act on single operand to perform an operation.
Operation | Operator |
---|---|
Positive | + variable |
Negation | – variable |
Prefix Increment | ++ variable |
Prefix decrement | — variable |
Post fix increment | variable ++ |
Post fix decrement | variable — |
Logical Complement | ! |
Placing + or – before a variable changes or indicates whether the value is positive or negative.
Prefix and Post fix operators, increment or decrement a variable value by 1. Prefix will increment/decrement before returning the value. Post fix will return value first then proceed to increment/decrement.
Positive Operation
Numbers are by default positive, so placing a + sign before a number doesn’t make sense.
Negation
int n2 = -10; // n2 will be -10
int n3 = -n1; // n3 will be -10
Prefix Increment/decrement
n1 = 1;
++n1; // n1 will be 2
int n4 = ++n1;
System.out.println(n1 + " " + n4); // n1 will be 3, n4 will be 3
n4 = --n1;
System.out.println(n1 + " " + n4); // n1 will be 2, n4 will be 2
Post fix Increment / Decrement
n1 = 1;
n1++; // n1 will be 2
int n5 = n1++;
System.out.println(n1 + " " + n5); // n1 will be 3, n5 will be 2
n5 = n1--;
System.out.println(n1 + " " + n5); // n1 will be 2, n5 will be 3
Logical Compliment Operator
Logical Compliment Operator inverts the value of a boolean variable.
boolean isSuccess = !false; // true will be stored
Compound Assignment Operators
Compound assignment operation is short hand of performing an arithmetic operation on a variable and storing the result on the same variable itself.
Compound Assignment Operator | Usage (a is 6 for all scenarios) | Value of a | Equivalent |
---|---|---|---|
+= | a += 3 | 9 | a = a + 3 |
-= | a -= 3 | 3 | a = a – 3 |
*= | a *= 3 | 18 | a = a * 3 |
/= | a /= 3 | 2 | a = a / 3 |
%= | a %= 3 | 0 | a = a % 3 |
Bitwise Operators
Java provides Bitwise Logical and Shift operators and that can be used along with long, int, short, byte and char primitive data types.
Bitwise Logical Operators
Bitwise Logical Operators are AND (&), OR (|), XOR (^) and NOT (~).
Bit 1 | Bit 2 | Bit 1 & Bit 2 | Bit 1 | Bit 2 | Bit 1 ^ Bit 2 | ~Bit 1 |
---|---|---|---|---|---|
1 | 1 | 1 | 1 | 0 | 0 |
1 | 0 | 0 | 1 | 1 | 0 |
0 | 1 | 0 | 1 | 1 | 1 |
0 | 0 | 0 | 0 | 0 | 1 |
AND – & Operator
AND operation will result in 1 only when it is performed between two bits whose values are 1. If either of the two is 0, the result will be 0.
2 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
4 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 |
2 & 4 = 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
2 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
3 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 1 |
2 & 3 = 2 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
package com.techstackjournal;
public class ANDOperator {
public static void main(String[] args) {
System.out.println(2 & 4); // 0
System.out.println(2 & 3); // 2
}
}
OR – | Operator
When OR Operation is performed between bits of two operands, it will result in 1, when either or both of those bits are 1. If both bits are 0, then the result will be 0.
2 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
4 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 |
2 | 4 = 6 | 0 | 0 | 0 | 0 | 0 | 1 | 1 | 0 |
package com.techstackjournal;
public class OROperator {
public static void main(String[] args) {
System.out.println(2 | 4); // 6
}
}
XOR – ^ Operator
In XOR Operation, the result will be 1 only when one of the 2 bits is 1. Even if both bits are 1 the result will be 0.
2 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
6 | 0 | 0 | 0 | 0 | 0 | 1 | 1 | 0 |
2 ^ 6 = 4 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 |
package com.techstackjournal;
public class XOROperator {
public static void main(String[] args) {
System.out.println(2 ^ 6); // 4
}
}
NOT or Compliment – ~ Operator
NOT Operator is applied on a single variable. When it is applied all of its bits will be inverted, that is, 0s will become 1s, and 1s will be 0s.
6 = 00000000000000000000000000000110
~6 = 11111111111111111111111111111001 = -7
package com.techstackjournal;
public class NOTOperator {
public static void main(String[] args) {
System.out.println(~6);
}
}
Bitwise Shift Operators
There are primarily 3 bitwise shift operators, left shift (<<), signed right shift (>>) and unsigned right shift (>>>). The left-hand operand is the value to be shifted and the right-hand operand specifies the shift distance to be shifted.
Left Shift – << Operator
In the below table, we are left shifting 2 by 1 bit, which becomes 4.
2 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
2 << 1 = 4 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 |
package com.techstackjournal;
public class LeftShiftOperator {
public static void main(String[] args) {
System.out.println(2 << 1); // Output will be 4
}
}
Signed Right Shift – >> Operator
In the below table, we are right shifting 4 by 1 bit, which becomes 2.
4 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 |
4 >> 1 = 2 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
package com.techstackjournal;
public class RightShiftOperator {
public static void main(String[] args) {
System.out.println(4 >> 1); // Output will be 2
}
}
In the below program, we are shifting bits of a negative number. After shifting the bits by 1, it fills the empty spaces in the left with 1.
-2 = 11111111111111111111111111111110
-2 >> 1 = 11111111111111111111111111111111 = -1
package com.techstackjournal;
public class LeftShiftOperator {
public static void main(String[] args) {
System.out.println(-2 >> 1);
}
}
Unsigned Right Shift – >>> Operator
Unsigned Right Shifting is similar to that of signed right shifting. The only difference is, if left-hand operand is negative, the expression will be evaluated as (n >> s) + (2 << ~s). Due to this, after all bits are shifted right, the empty spaces will be filled with zero.
-2 = 11111111111111111111111111111110
-2 >>> 1 = 01111111111111111111111111111111 = 2147483647
package com.techstackjournal;
public class UnsignedRightShiftOperator {
public static void main(String[] args) {
System.out.println(-2 >>> 1); // Output will be 2147483647
}
}
Equality and Relational Operators
Equality and Relational operators are used for comparing two operands and return a boolean value (true / false). These operators can be used on all primitive data variables.
Equals ( == ) Operator
== Operator compares primitive values of two operands to check if both are equal or not and return boolean value result.
int num1 = 5;
int num2 = 10;
System.out.println(num1 == num2); // false will be stored in result
Not Equals ( != ) Operator
Operator != compares primitive values of two operands to check inequality between them.
int num1 = 5;
int num2 = 10;
System.out.println(num1 != num2); // true will be stored in result
Greater Than ( > ) Operator
Compares if left operand is greater than right operand.
int num1 = 5;
int num2 = 10;
System.out.println(num1 > num2); // prints false
Greater Than or Equal ( >= ) Operator
Compares if left operand is greater than or equals to right operand.
int num1 = 5;
int num2 = 5;
System.out.println(num1 >= num2); // prints true
Less Than ( < ) Operator
Compares if left operand is less than right operand, if yes, returns true, else returns false.
int num1 = 5;
int num2 = 10;
System.out.println(num1 < num2); // prints true
Less Than or Equal ( <= ) Operator
Compares if left operand is less than or equals to right operand, if yes, it returns true, else it returns false.
int num1 = 5;
int num2 = 5;
System.out.println(num1 <= num2); // prints true
Logical Operators
Logical Operators and Conditional Operators are used for combining multiple conditions together.
Operators &, | and ^ are called Logical Operators when they are used with along with boolean expressions. Logical Operators evaluate all conditions before performing AND operation on them. These operators can be better explained with their truth tables.
Truth Table – AND (&)
Expression 1 | Expression 2 | Result |
---|---|---|
true | true | true |
true | false | false |
false | true | false |
false | false | false |
Truth Table – OR (|)
Expression 1 | Expression 2 | Result |
---|---|---|
true | true | true |
true | false | true |
false | true | true |
false | false | false |
Truth Table – XOR (^)
Expression 1 | Expression 2 | Result |
---|---|---|
true | true | false |
true | false | true |
false | true | true |
false | false | false |
Logical Operators Example:
package com.techstackjournal;
public class LogicalOperators {
public static void main(String[] args) {
System.out.println(2 > 1 & 2 < 3); // true & true = true
System.out.println(2 > 1 | 2 > 0); // true | true = true
System.out.println(2 == 2 ^ 1 == 1); // true ^ true = false
}
}
Conditional Operators
Operators && and || are called as Conditional Operators. Conditional Operators are similar to Logical Operators, with one exception, that is, right hand side expressions will be evaluated only if left hand expressions are not enough to determine the result.
Truth Table – AND (&&)
Expression 1 | Expression 2 | Result |
---|---|---|
true | true | true |
true | false | false |
false | not evaluated | false |
false | not evaluated | false |
Truth Table – OR (||)
Expression 1 | Expression 2 | Result |
---|---|---|
true | not evaluated | true |
true | not evaluated | true |
false | true | true |
false | false | false |
Conditional Operators Example:
package com.techstackjournal;
public class ConditionalOperators {
public static void main(String[] args) {
System.out.println(2 > 1 && 2 < 3); // true && true = false
System.out.println(2 > 3 && 3 < 5); // false && - = false
System.out.println(2 > 3 || 2 > 0); // false || true = true
System.out.println(2 > 1 || 4 > 2); // true || - = true
}
}
In the above program, expressions 3 < 5 and 4 > 2 will never be executed, they are dead code.
Ternary Operators ( ? : )
Ternary Operators can be used when we want to initialize a variable on the basis of a condition. Ternary operation has 3 parts, 1) boolean expression 2) success expression (3) failure expression.
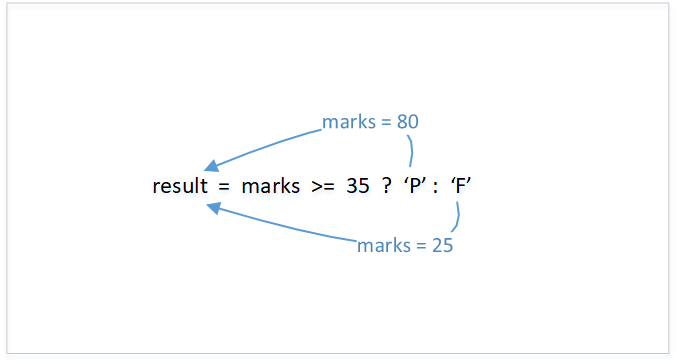
Here, boolean expression is, marks >= 35
. If this condition is true
, success expression will return ‘P’ to result. If this condition is false
, failure expression will return ‘F’ to result.
Ternary Operator Example
package com.techstackjournal;
public class TernaryOperator {
public static void main(String[] args) {
char result;
int marks = 50;
result = marks >= 35 ? 'P' : 'F';
System.out.println("Student Exam Status: " + result);
}
}
Student Exam Status: P