In this example, we will see how to reverse an integer in Java using mathematical equations, StringBuffer and StringBuilder classes.
Reverse an Integer in Java using Mathematical Equations
To reverse an integer mathematically, first thing what we should do is to take out the last digit as a separate integer. That is possible by dividing the number by 10.
After dividing any integer with 10, we get reminder and that reminder will be the last digit of the source number. Quotient will be the remaining part of the number without the last digit.
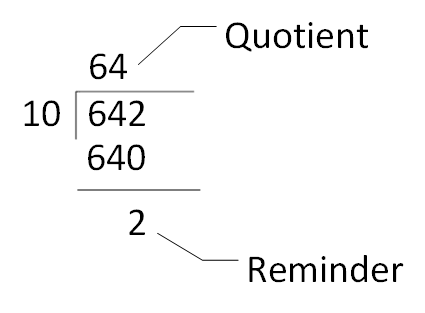
Once we split the source number, we should repeat the same process to pull out another last digit from source and append the second last digit mathematically to the last digit we already have pulled.
To append to digits together mathematically, we can multiple the first digit with 10 and add the second digit to the result. For example, we want to append 2 and 6 together mathematically. To do that, I can write the formula as 2 * 10 + 6, which will get me 26.
We should repeat this process multiple times, that is taking out the last digit and appending it to the result, till there are not digits left to pull out from the source number.
package com.techstackjournal;public class ReverseNumber { public static void main(String[] args) { int num = 642; int reverse = 0; while (num > 0) { reverse = reverse * 10 + num % 10; num = num / 10; } System.out.println(reverse); }}
Output:
246
Dry Run:
Iteration | num | reverse | num%10 (A) | reverse*10 (B) | reverse (A+B) | num/10 |
---|---|---|---|---|---|---|
1 | 642 | 0 | 2 | 0 | 2 | 64 |
2 | 64 | 2 | 4 | 20 | 24 | 6 |
3 | 6 | 24 | 6 | 240 | 246 | 0 |
Explanation
This entire program will take 3 iterations, our dry run table proves that. Why only 3 iterations, because we only have 3 digits in num
variable. With each iteration, we are taking out the last digit from num
. In the last iteration, after taking out the last digit from source number num
, it will not have anymore digits in it, so its value will be 0. So, when num
becomes 0 we should exit the loop.
As we discussed earlier, to get the last digit, we need to find the reminder of the division of source number with 10. A modulus operator % divides a number with another number but returns the reminder.
Once we calculate the last digit, we need to append this to previous last digit. But for the first iteration, there will not be any value, so it will be 0. So, for the first iteration, reverse * 10 will be zero, as reverse itself is zero.
After last digit appended to its previous last digit, we also need to change the source number num by removing its last digit from it. We are doing it by dividing it by 10 and storing the result in itself.
Reverse an Integer using StringBuffer
In this example, we’ll be using StringBuffer
class to reverse the integer. StringBuffer
has a built-in method that can reverse a text. To reverse an integer, we’ll be creating a StringBuffer
object and append the integer that we wish to reverse. Once we create the StringBuffer
this way, then we can call the reverse
method on StringBuffer
object to return reversed number. But this reversed number will be still StringBuffer
. To get the reversed number as integer, we’ll have to first convert it to a String
object by calling toString()
method on StringBuffer
object. Once we got the String
object, we then use Integer.parseInt
to get integer value of that String
object.
package com.techstackjournal;
public class ReverseNumber {
public static void main(String[] args) {
int num = 642;
int reverse = 0;
StringBuffer sb = new StringBuffer();
sb.append(num);
String str = sb.reverse().toString();
reverse = Integer.parseInt(str);
System.out.println(reverse);
}
}
Reverse an Integer using StringBuilder
Reversing an integer using StringBuilder is no difference from StringBuffer implementation, so it doesn’t require much explanation.
package com.techstackjournal;
public class ReverseNumber {
public static void main(String[] args) {
int num = 642;
int reverse = 0;
StringBuilder sb = new StringBuilder();
sb.append(num);
String str = sb.reverse().toString();
reverse = Integer.parseInt(str);
System.out.println(reverse);
}
}